Crafting GUIs
Virtual controls and visualisations for your project
Bela has an integration for creating GUIs, right in the IDE. This means you can make graphical interfaces to send data to your Bela system, make visuals that are controlled by your Bela system, or both! This enables lots of new possibilities, from visuals for multi-media performances to generating input for your Bela system from on-screen sliders. The Bela GUI integration uses p5.js to generate on-screen graphics, and works with both C++ and Pure Data projects.
This article details the Bela GUI integration, and provides an example of how to use it.
Table of contents
- The Bela GUI library: Passing data around
- Bela + p5.js =
- Launching the GUI
- The structure of a project with a GUI
- Some GUI examples
- Adding a GUI to a project
- Learn more
The Bela GUI library: Passing data around
The Bela GUI library allows you to pass data from your Bela project to a graphical interface, or from a graphical interface to your Bela system. This means that you could control on-screen visuals with generated signals or sensor input, or control your Bela’s audio output with what you do with an on-screen interface. Best of all, the data doesn’t have to to only go in one direction - it can travel in both.
Bela + p5.js =
Bela’s GUI functionality works with p5.js, a JavaScript library for creating powerful and dynamic audiovisuals in the browser. It’s very similar to Processing, a common audio-visual programming environment used extensively by artists. Since Bela is geared towards people who make creative things with technology, we chose p5.js as it’s already widely used in the creative maker community.
Launching the GUI
When your project is running, your GUI is ready to launch. Click the Launch GUI
button in the toolbar:
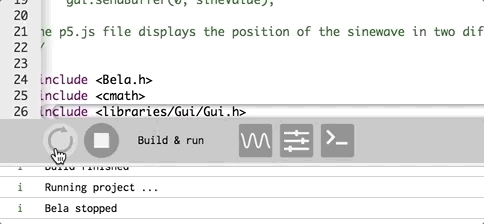
The GUI will open in a new tab.
The structure of a project with a GUI
In addition to your core project files, when you have a GUI you will also have a file which is called sketch.js
as part of your project. This is where the p5.js code for the GUI is stored.
Some GUI examples
Sending data from Bela to the GUI
Before we go any further, you can check out the GUI in action.
C++: Ambient ellipse
In the
Examples tab, go to GUI > ambient-ellipse
. Run the project and launch the GUI. You’ll see this:
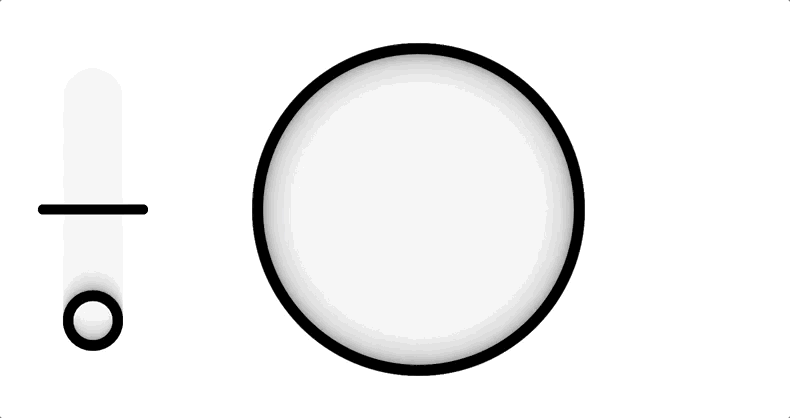
In our render.cpp
file we generate a low frequency sine wave, and send it to the GUI with the gui.sendBuffer()
function. In the sketch.js
file we’re receiving this value with Bela.data.buffers[0]
, and using that value to control the position of the ellipse on the side, and the size of the central ellipse.
Pure Data: Shimmering rings
In the
Examples tab, go to PureData > gui-send
. Run the project and launch the GUI. You’ll see this:
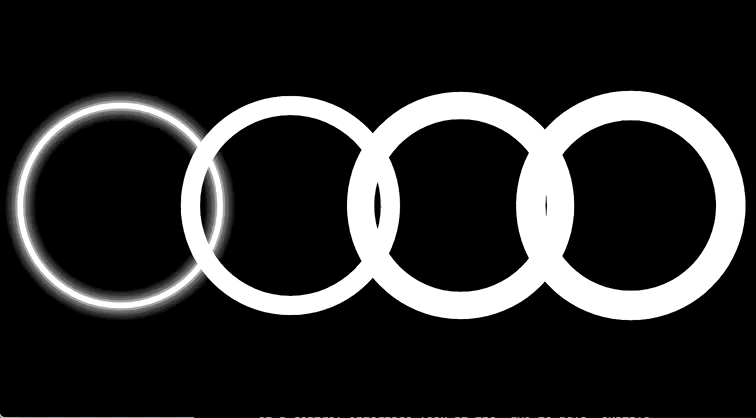
In the _main.pd
sketch we’ve created four oscillators. We group these together using the [pack]
object, and send that to the GUI with [s bela_guiOut]
. In the sketch.js
file we receive this group of values with Bela.data.buffers[0]
and loop through those values, and use them for rendering each of the four rings.
Sending data from the GUI to Bela
As well as sending data from Bela to the GUI, we can also send data from the GUI to control the behaviour of Bela.
C++: Gui to Bela
In the
Examples tab, go to GUI > gui-to-bela
. Run the project and launch the GUI. Make sure you have your headphones plugged into Bela’s audio output. You’ll see this:
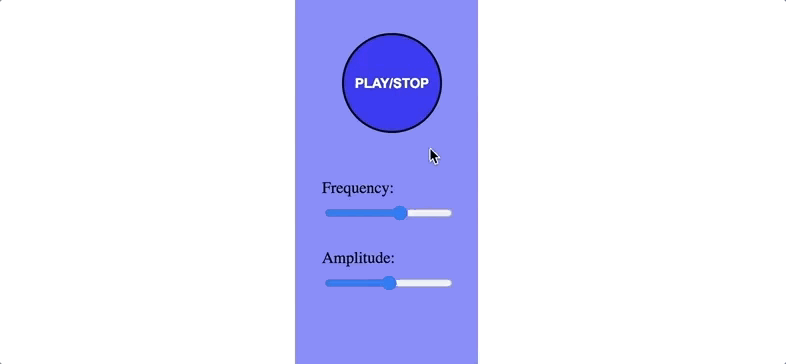
By adjusting the sliders you can adjust the frequency and amplitude of the sine wave being generated on Bela. You can also stop and start the playback by clicking the button.
In sketch.js
the variable buffer
holds three values - frequency, amplitude, and the button state. These are set in each iteration of the draw()
function and sent to Bela with Bela.data.sendBuffer()
. We receive these in render.cpp
with gui.getDataBuffer()
, and applied to the frequency, amplitude and playback of the sine signal in the render()
function.
Pure Data: Mouse sounds
In the
Examples tab, go to Pure Data > gui-receive
. Run the project and launch the GUI. Make sure you have your headphones plugged into Bela’s audio output. You’ll see this:
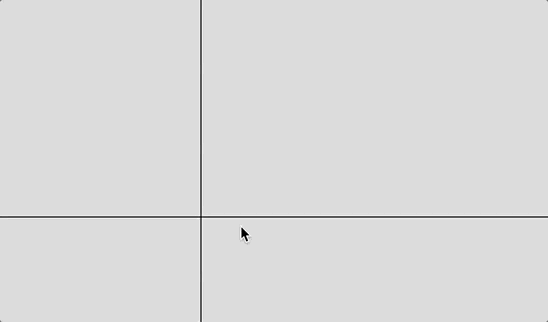
By moving your mouse around the screen you will control the frequency (x axis) and amplitude (y axis) of the sine signal generated by Bela. In sketch.js
we capture the X and Y position of the mouse, and send them to Bela with Bela.data.sendBuffer(0, 'float', [mouseX, mouseY])
. Then in _main.pd
we receive these values with [r bela_guiControl]
and apply them to the oscillator.
Adding a GUI to a project
C++
A GUI can be added to any Bela C++ project by adding three lines to the render.cpp
file and adding a sketch.js
file.
First, include the GUI library in your render.cpp
file. Go to the
Libraries tab. Expand the Gui > Include this library
tab and copy the include statement. Paste this at the top of your render.cpp
file.
Next, after the #include
statements at the top, create a GUI object like this:
Gui gui;
Then, in your setup()
function we need to get ready to send and receive data from the GUI. Include this line in setup()
:
gui.setup(context->projectName);
Finally, create your sketch.js
file. In the
Project Explorer tab choose click the Create new file
icon. In the dialog box, name it sketch.js
.
Pure Data
You don’t need to do anything to your _main.pd
sketch, just create a sketch.js
file.
In the
Project Explorer tab choose click the Create new file
icon. In the dialog box, name it sketch.js
.
Structuring sketch.js
Whether you’re working in C++ or Pure Data, your sketch.js
is a p5.js file and should have the structure of any p5 sketch:
function setup() { // This function runs once at the beginning of the sketch
}
function draw() { // This function repeats over and over as the sketch runs
}
sketch.js
is a p5.js file, so remember that we're writing in Javascript, not C++!
Learn more
p5.js Reference
The p5.js site is a great place to learn how to create graphics with p5. It also has a very extensive code reference.
p5js Editor
p5js also has a browser-based editor, so you can experiment with creating graphics and see the results instantly. If you sign up for an account you can also save all your experiments online.
The Bela GUI examples
The Examples tab of the Bela IDE has a GUI section that contains lots of C++ examples. Find GUI examples in Pd in the Pure Data section.